Javascript For Each Loop
JavaScript forEach
is one of the built-in methods you can use to loop through the array and execute some functionality on each item without returning a new array or mutating the original one.
The forEach
method accepts a callback function, and optionally, we can pass thisArg
(which will be used as this when executing the callback). The callback or provided function is called once on each item in an array in ascending order.
const animals = ['Cat', 'Dog', 'Bird'];
// We're passing an anonymous function as a callback to forEach
animals.forEach(function (animal) {
if (animal !== 'Bird') console.log(animal);
});
// -- ๐ console.log output --
// "Cat"
// "Dog"
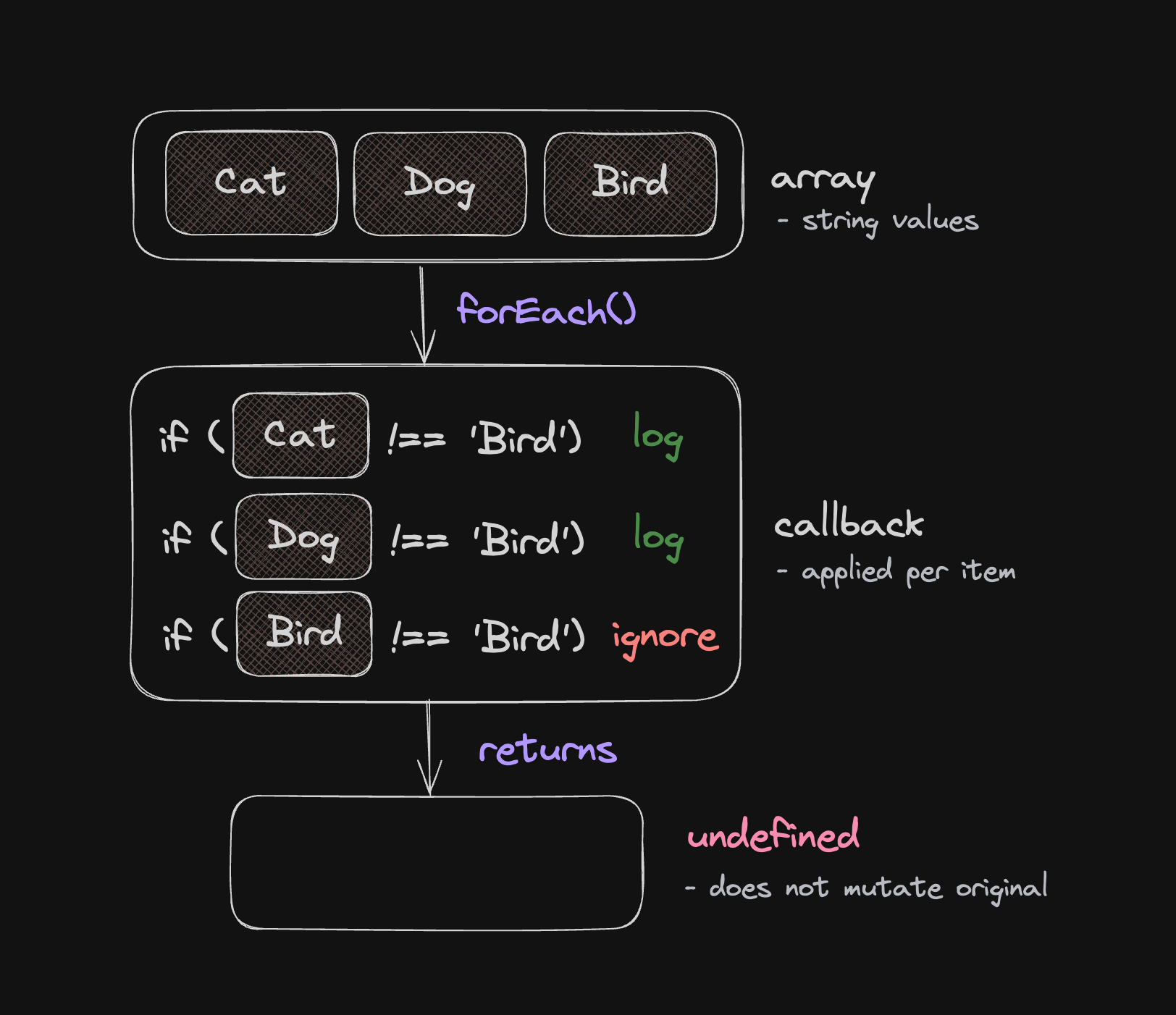
You can also rewrite the anonymous function if you want to use the ES6 arrow syntax. This would make it a one-liner:
animals.forEach((animal) => animal !== 'Bird' ? console.log(animal) : undefined);
The callback or anonymous function we're passing to forEach
method has two more optional parameters. The first of the optional parameters is the index.
const animals = ['Cat', 'Dog', 'Bird'];
animals.forEach(function (animal, index) {
if (index === 0) console.log(animal);
})
// -- ๐ console.log output --
// "Cat"
Looping through the array-like object are topics on their own but in a nutshell, if you're working in a browser environment with JavaScript, the most common array-like object you probably encounter is a NodeList. Example is the result of document.querySelectorAll('p')
.
Some built-in array methods such as filter or map cannot be used on array-like objects or NodeList directly and require conversion to a regular array. However, the forEach
method can be directly called on the NodeList.
Finally, the use case of the forEach
method might be to aggregate some values:
const items = [{ price: 100 }, { price: 50 }, { price: 10 }];
let sum = 0;
items.forEach((item) => {
sum += item.price;
});
console.log(sum);
// -- ๐ console.log output --
// 160
Sure, for this example we might stick to a functional approach and use the reduce
method. However, if you need to aggregate more than one value, it might be more readable to use the forEach
method.
When to use forEach
method?
Logging: As shown in the examples above, you can use the method for conditional logging based on some criteria. This is effective for debugging.
Executing side-effects: Since
forEach
method does not modify an array nor return any output, it might be useful for calling non-returning functions on certain items of an array.Aggregating values: Another use case might be to aggregate values from the array.
Considerations:
The
forEach
method does not modify the original array unless it contains objects on which you mutate the properties โ I do not recommend doing mutations this way and instead use themap
method for such use case.It always returns
undefined
, which means you never want assign the result to a variable. Even if you would, it would be just undefined, no matter what callback you pass to it.Unlike
map
orfilter
,forEach
is generally used for operations that involve side-effects.Unlike the native for loop, you cannot break the
forEach
loop. Thereturn
keyword would only apply to its scope, which is a callback, instead of a method and thus won't stop the loop.