Javascript Filter - How to use array filter method?
JavaScript filter is another one of the essential methods you can use to loop through the array. The output of this method is a shallow copy of the provided array without the items you filtered out.
The filter method accepts a callback function, and optionally, we can pass thisArg
(which will be used as this when executing the callback). The callback or provided function is called once on each item in an array in ascending order.
const animals = ['Cat', 'Dog', 'Bird'];
// We passed an anonymous function as a callback to filter
const out = animals.filter(function (animal) {
return animal !== 'Bird';
});
console.log(out)
// -- ๐ console.log output --
// Array(2) ["Cat", "Dog"]
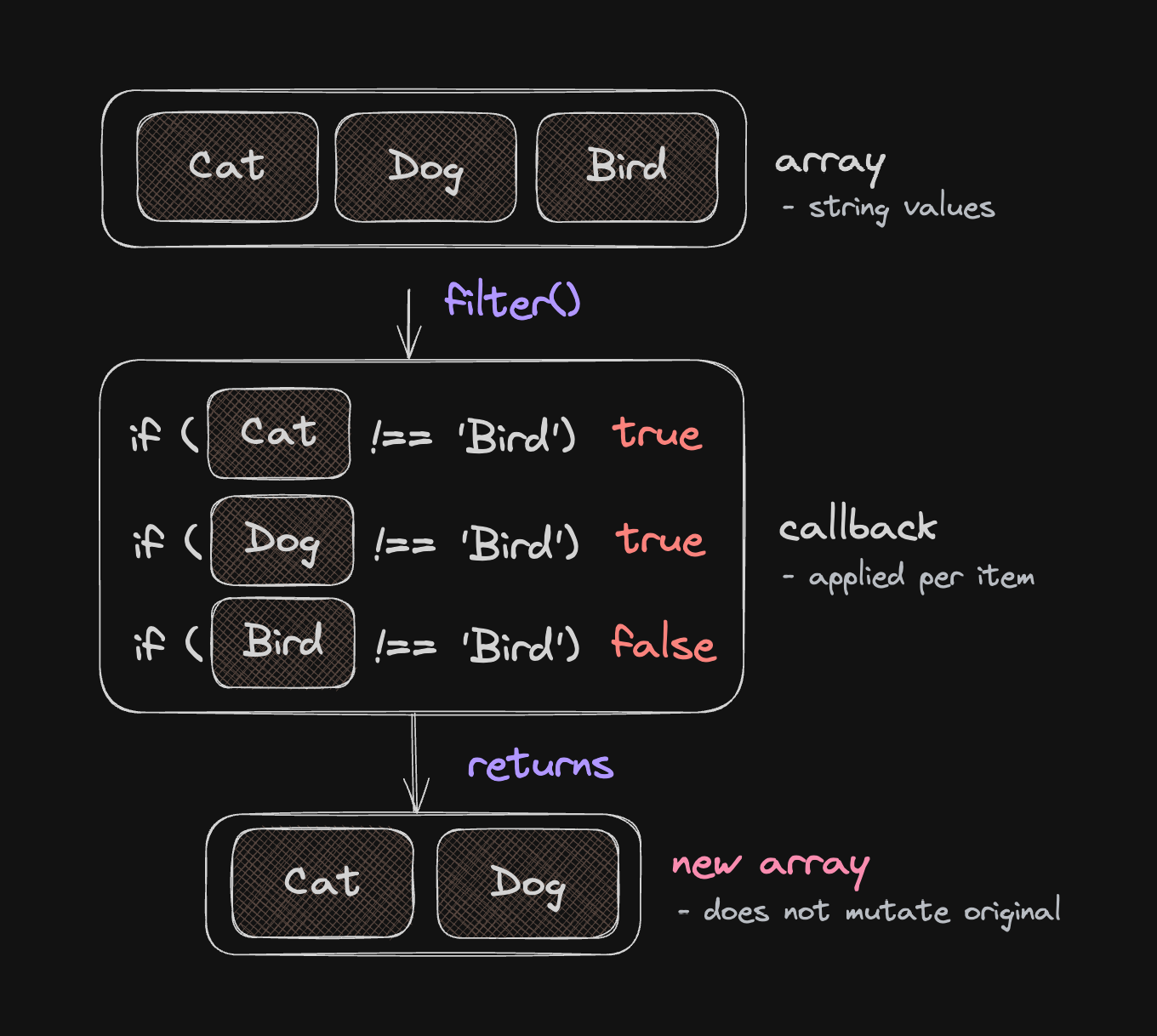
You can also rewrite the anonymous function if you want to use the ES6 arrow syntax. This would make it a one-liner:
const out = animals.filter((animal) => animal !== 'Bird');
Although not as common, we can declare the callback as non-anonymous function. It's useful if the callback needs to be reused in our code.
const animals = ['Cat', 'Dog', 'Bird', 'Ant', 'Spider'];
// This function probably won't be needed anywhere else
// but let's pretend it would be
function containsLetterA(animal) {
return animal.toLowerCase().includes('a');
}
const out = animals.filter(containsLetterA);
// โ๏ธ is shorthand of:
// animals.filter((animal) => containsLetterA(animal));
console.log(out)
// -- ๐ console.log output --
/* Array(3) ["Cat", "Ant"]
*/
JavaScript executes the callback once for every item in the array. There is no difference if we use callback (named function) or anonymous function. In the last example, iterated item (in our case avenger) is passed as a first parameter to the callback implicitly.
Callback or anonymous function we pass to filter has two more optional parameters. First of the optional parameters is the index.
const animals = ['Cat', 'Dog', 'Bird'];
const out = animals.filter(function (animal, index) {
return index === 0;
});
console.log(out)
// -- ๐ console.log output --
/* Array(3) ['Cat']
*/
Array-like object are topics on their own, so let's just jump on the examples. If you're working in a browser environment with JavaScript, the most common array-like object you probably encounter is a NodeList - you can get it by trying document.querySelectorAll('p')
. You may choose to get different element, not necessarily a paragraph.
You can't use filter on NodeList as it would throw Uncaught TypeError: filter is not a function. We need to first convert/copy the array-like object to the array. In the following example, we can see an array-like object:
const animalsInArrayLikeObject = {
"0": "Cat",
"1": "Dog",
"2": "Bird",
"length": 3
};
Now in order to filter the animalsInArrayLikeObject
, we can't call filter directly. It would throw the same error as with NodeList. We have couple of options though:
// Array-like object on which we can't use (call) filter
// It would throw 'filter is not a function' TypeError
const animalsInArrayLikeObject = {
"0": "Cat",
"1": "Dog",
"2": "Bird",
"length": 3
};
// Named function (callback) called 'fn'
function fn(animal, index) {
return index === 0;
}
// Create new shallow copy from an array-like object
// Array.from returns an Array instance
const out1 = Array.from(animalsInArrayLikeObject).filter(fn);
// We can call slice method
const out2 = Array.prototype.slice
.call(animalsInArrayLikeObject).filter(fn);
// We can call filter indirectly by using a call method
const out3 = Array.prototype.filter
.call(animalsInArrayLikeObject, fn);
The filter array method is extremely useful to explicitly filter out items from an array. You can also put side-effects in the callback you pass to filter but keep in mind that under normal circumstances you can't break the iteration.
I'll link these as soon as I cover them in the separate article. Consider reading up on them, as if you don't you may end up reinventing a method that already exists. For instance some and every are not as well-known as the other array methods.